Use Environment Variables in Next.js
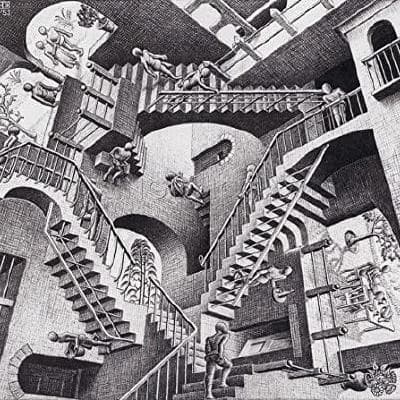
During the week, I was working on the new project. In development process, I simply directly connected Vercel Postgres with Prisma in Next.js. However, the Hobby plan of Vercel Postgres has usage limits and I almost exceeded the data transfer limits. To save the amount of data transfer, I have to connect local Postgres with the project for testing and debuging. So I think I should use environment variables to distinguish between the development and production environments automatically.
NODE_ENV
As the documentation said, if the environment variable NODE_ENV
is unassigned, Next.js automatically assigns development when running the next dev
command, or production for all other commands.
Do not set
NODE_ENV
in.env
or.env.*
, it may cause non-standard environment errors [https://nextjs.org/docs/messages/non-standard-node-env]
.env*
Files and Environment VariablesYou also can create a .env.development
for development environment and add local PostgreSQL variable in it.
# Postgres
POSTGRES_URL="postgresql://postgres:xxx@localhost:5432/postgres"
POSTGRES_PRISMA_URL="postgresql://postgres:xxx@localhost:5432/postgres?pgbouncer=true&connect_timeout=15"
POSTGRES_URL_NON_POOLING="postgresql://postgres:xxx@localhost:5432/postgres"
POSTGRES_USER="postgres"
POSTGRES_HOST="localhost"
POSTGRES_PASSWORD="xxx"
POSTGRES_DATABASE="postgres"
And create a .env.production
for production environment and add Vercel Postgres variable in it.
# Postgres
POSTGRES_URL="************"
POSTGRES_PRISMA_URL="************"
POSTGRES_URL_NON_POOLING="************"
POSTGRES_USER="************"
POSTGRES_HOST="************"
POSTGRES_PASSWORD="************"
POSTGRES_DATABASE="************"
After that, when running next dev
, the Next.js project will automatically load environment variables from .env.development
.
Or if deploying Next.js project to production environment, it will loading production environment variables from .env.production
by default.
You can also add the environment variables shared between development and production environments in .env
file, which will be loaded wherever the environments are.
# Postgres
NEXT_PUBLIC_IMAGES_URI = **********
NEXT_PUBLIC_ICON_URL = **********
Development Mode: (bun dev): .env.development.local
> .env.local
> .env.development
> .env
Production Mode: (bun run build): .env.production.local
> .env.local
> .env.production
> .env
Now it will not waste the usage limits of Vercel Postgres when developing project in local.